Accessibility: Bold Text with custom fonts
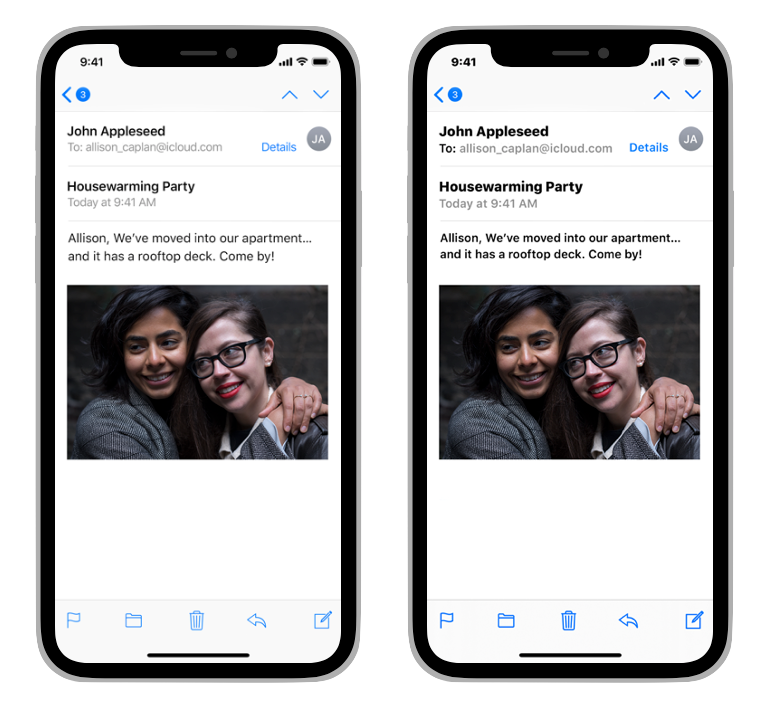
Apple guidelines
As Apple is stating in its guidelines:
Ensure that your app responds correctly and looks good when users enable bold text. People turn on the bold text accessibility setting to make text and glyphs easier to see. In response, your app should make all text bolder and give all glyphs an increased stroke weight.
Using custom fonts, we need to add support for Bold Text functionality. It can be enabled by the user on his phone via Settings โ Accessibility โ Display & Text Size โ Bold Text.
Statistics
How many users are using Bold Text Accessibility functionality?
In the Immoweb iOS app, it has been over a year since we started recording statistics about which accessibility features are used by our users.
Immoweb is one of the biggest Belgian apps (and is mostly used in Belgium)
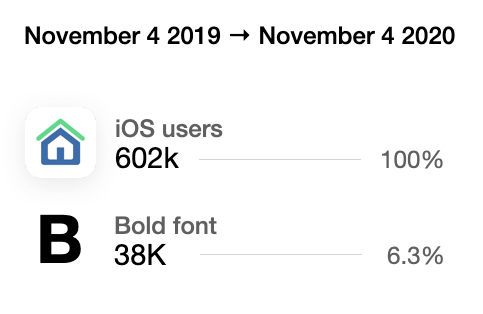
If we look specifically at users using the Bold Text functionality, we can see it represents 6.3% of our audience which is not negligible.
Solution
In our previous article, we extended UIFont
with 2 static functions to build fonts based on our custom FontStyle
s. It looked like this:
extension UIFont {
/// Returns a font matching `style`
static func font(style: <ModuleName>.TextStyle) -> UIFont {
var descriptor = UIFontDescriptor(name: style.name, size: style.size)
if let symbolicTraits = style.emphasis.symbolicTraits {
descriptor = descriptor.withSymbolicTraits(symbolicTraits)!
}
return UIFont(descriptor: descriptor, size: style.size)
}
/// Returns a font matching `style` scaled to the current Content Size Category.
static func scaledFont(style: <ModuleName>.TextStyle) -> UIFont {
UIFontMetrics.default.scaledFont(for: font(style: style))
}
}
We can iterate on this solution to support bold fonts:
extension UIFont {
/// Returns a font matching `style`
static func font(style: <ModuleName>.TextStyle) -> UIFont {
var descriptor = UIFontDescriptor(name: style.name, size: style.size)
if let symbolicTraits = style.emphasis.symbolicTraits {
descriptor = descriptor.withSymbolicTraits(symbolicTraits)!
}
if UIAccessibility.isBoldTextEnabled {
descriptor = descriptor.withSymbolicTraits(.traitBold)!
}
return UIFont(descriptor: descriptor, size: style.size)
}
/// Returns a font matching `style` scaled to the current Content Size Category.
static func scaledFont(style: <ModuleName>.TextStyle) -> UIFont {
UIFontMetrics.default.scaledFont(for: font(style: style))
}
}
Here we are:
- Checking if Bold Text functionality is enabled through
UIAccessibility.isBoldTextEnabled
variable (note that since iOS 13 you can also checkUITraitCollection.legibilityWeight
) - Adding the
.traitBold
trait to our fontdescriptor
Going further
If we take another look at the example provided by Apple we can see that even bold texts are getting bolder.
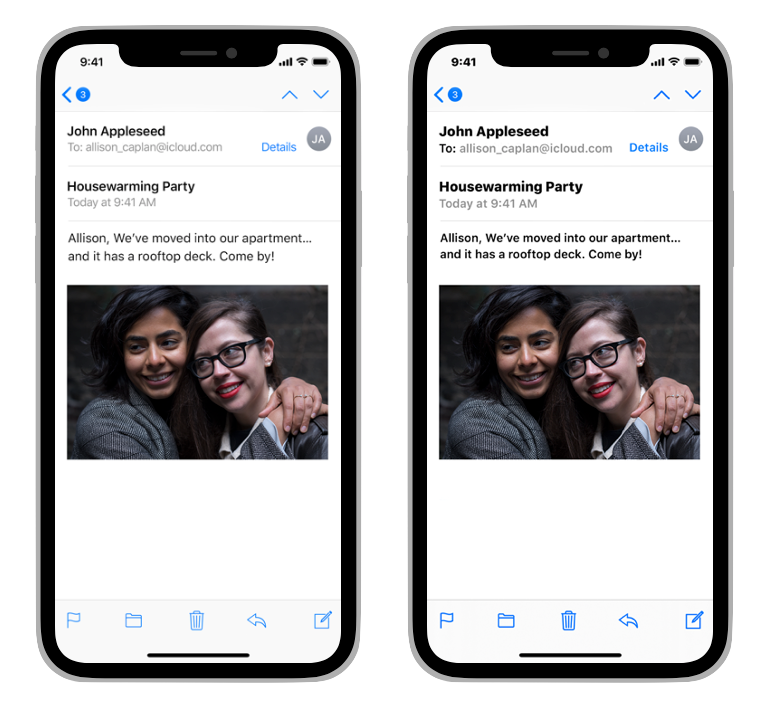
The problem with our solution above is that it will set regular fonts to boldbut bold fonts will stay bold. While they should become black (if your custom font supports this variant, of course).
A way we could handle this is by mapping each font to its bold equivalent. For example:
- Montserrat-Regular โ Montserrat-Bold
- Montserrat-Italic โ Montserrat-BoldItalic
- Montserrat-Bold โ Montserrat-Black
- Montserrat-BoldItalic โ Montserrat-BlackItalic
Thank you for reading.
Together, weโll make Accessibility great again! ๐ช
Resources
- Previous article โ Dynamic Type: Scaling Custom Fonts
- Text size and weight โ Apple Human Interface Guidelines
Other articles of the โDynamic Typeโ series
Special thanks to Vincent Martin for proofreading this article ๐